AI Email Writer!
Meet AI Email Writer: The secret weapon for flawless emails! Craft clear, compelling messages in seconds with our cutting-edge AI. Try AI Email Writer and transform your communication today!
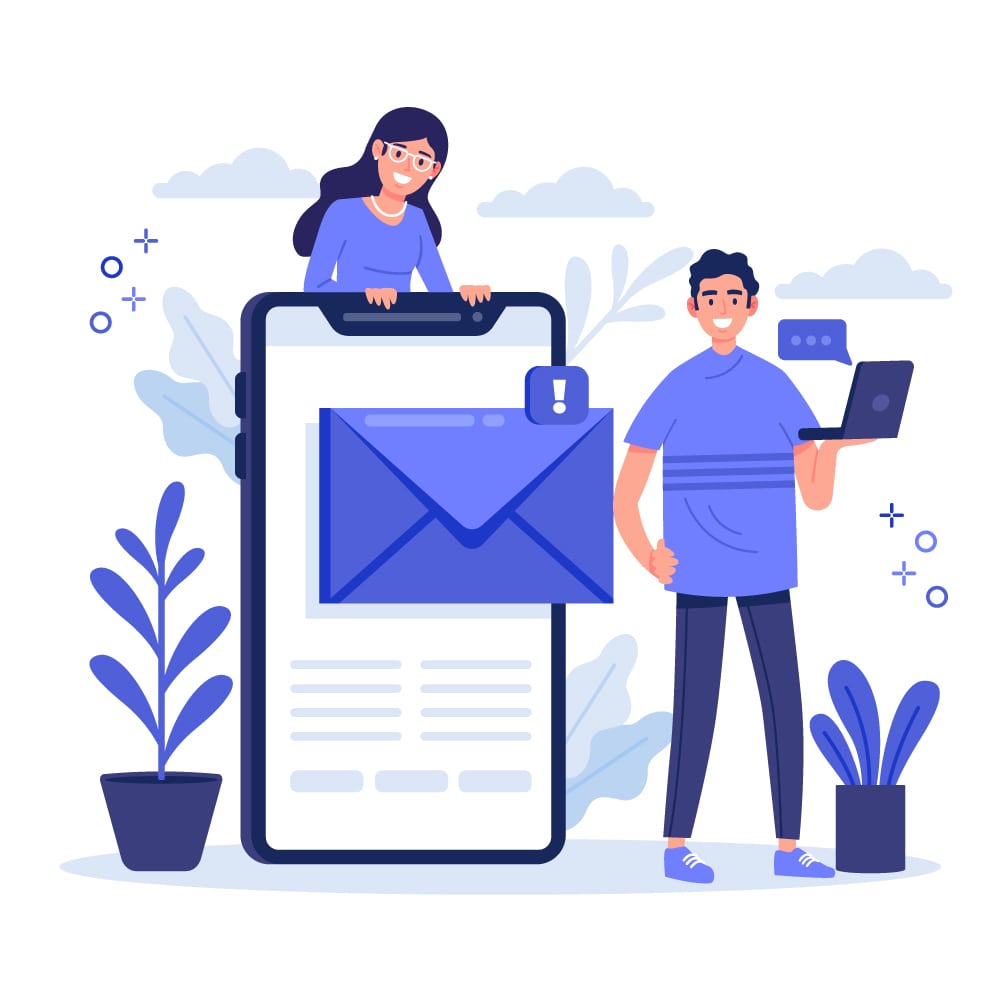
How It Works?
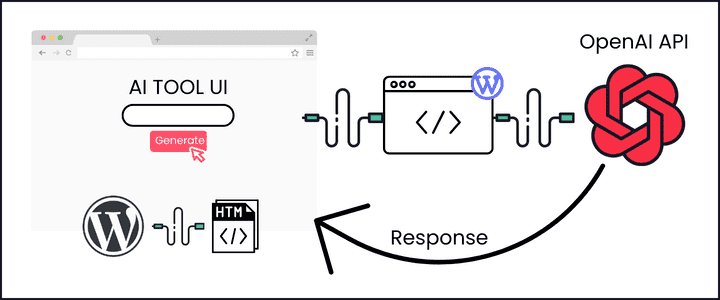
Back-End Codes
function openai_generate_text() {
// Get the topic from the AJAX request
$prompt = $_POST['prompt'];
// OpenAI API URL and key
$api_url = 'https://api.openai.com/v1/chat/completions';
$api_key = 'sk-XXX'; // Replace with your actual OpenAI API key
// Headers for the OpenAI API
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer ' . $api_key
];
// Body for the OpenAI API
$body = [
'model' => 'gpt-3.5-turbo',
'messages' => [['role' => 'user', 'content' => $prompt]],
'temperature' => 0.7
];
// Args for the WordPress HTTP API
$args = [
'method' => 'POST',
'headers' => $headers,
'body' => json_encode($body),
'timeout' => 120
];
// Send the request
$response = wp_remote_request($api_url, $args);
// Handle the response
if (is_wp_error($response)) {
$error_message = $response->get_error_message();
wp_send_json_error("Something went wrong: $error_message");
} else {
$body = wp_remote_retrieve_body($response);
$data = json_decode($body, true);
if (json_last_error() !== JSON_ERROR_NONE) {
wp_send_json_error('Invalid JSON in API response');
} elseif (!isset($data['choices'])) {
wp_send_json_error('API request failed. Response: ' . $body);
} else {
wp_send_json_success($data);
}
}
// Always die in functions echoing AJAX content
wp_die();
}
add_action('wp_ajax_openai_generate_text', 'openai_generate_text');
add_action('wp_ajax_nopriv_openai_generate_text', 'openai_generate_text');
Front-End Codes
Pros & Cons
✅Subject Line Analysis: Understands your input to create relevant email.
✅Quick Email Generation: Instantly produces a complete email with a single click.
✅Basic Grammar Check: Ensures the generated email is free from grammatical errors.
✅Simple Formatting: Applies basic formatting for a clean, readable email.
✅Basic Personalization: Adds the recipient’s name and other simple personal touches based on input.
❌Subscription Cost: The API key required for generating emails is paid.
❌Limited Customization: Offers minimal control over the email content and tone, which may not suit all needs.
❌Context Understanding: May lack the ability to fully grasp the context of the subject line.
❌Basic Features Only: Lacks advanced features like detailed grammar checking.
❌Dependence on Input Quality: Relies heavily on the quality and clarity of the subject line to generate an appropriate email.
Presented To:
The IT Department Of Superior University Sargodha Campus.
1. Prof, Shahid Ameer
2. Prof, Waseem Abaasi.
3. Prof, Ali Imran